First import the following namespaces:
using System.Linq;
using System.IO;
Then write the following code:
class Program { static void Main(string[] args) { string[] dirfiles = Directory.GetFiles("c:\\software\\"); var avg = dirfiles.Select(file => new FileInfo(file).Length).Average(); avg = Math.Round(avg/1000000, 1); Console.WriteLine("The Average file size is {0} MB", avg); Console.ReadLine(); } }
The code shown above uses the Average Extension method to compute the average of a sequence of' numeric values. The values in this case is the length of the files in the folder “c:/software”. The result is rounded off to one decimal place using Math.Round
OUTPUT
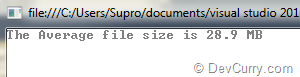
No comments:
Post a Comment